Add an owner to a Control
Add an owner to a control using the Vanta API
Assign an Owner to a Control
In this guide we'll cover how to assign an active user in Vanta as an owner of a Framework Control. Control owners are responsible for seeing the Control compliance through to completion. In most cases, the Vanta user will require access to view the Documents, Tests, and Policies associated with the Control they own.
Steps:
- GET The Control ID of the Control you'd like to assign an owner to via the List Controls endpoint.
- Alternatively from the Controls Page in Vanta.
- GET The User ID of the Vanta user you'd like to assign as an owner via the List People endpoint.
- Alternatively from the People Page in Vanta.
- POST The User ID of the new Control owner in the request body of the Set-Unset Control Owner endpoint.
List Controls Endpoint - Get your control id
Below we'll make a request to the List Controls endpoint to acquire our Control ID. This Endpoint can be filtered by Framework via the frameworkMatchesAny
query param. To filter by multiple frameworks, you can duplicate the frameworkMatchesAny
query param.
Endpoint:
/controls
Query Parameters:
-
pageSize (
<number>
): An integer value that specifies the number of resources to return per page. This parameter controls the size of each page in paginated responses. -
pageCursor (
<string>
): A string value used to navigate through pages of results. This parameter holds the cursor for the current page, allowing the API to fetch the next set of results. -
frameworkMatchesAny (
<string>
): Includes all controls belonging to one of the provided framework values in frameworkMatchesAny. This parameter is used to filter controls based on specified frameworks. Duplicate this param for each additional framework you're filtering by.
List Controls - Code Example
curl --location 'https://api.vanta.com/v1/controls?pageSize=10&pageCursor=%3Cstring%3E&frameworkMatchesAny=soc2' \
--header 'Accept: application/json' \
--header 'Authorization: Bearer vat_YOUR_TOKEN'
const myHeaders = new Headers();
myHeaders.append("Accept", "application/json");
myHeaders.append("Authorization", "Bearer vat_YOUR_TOKEN");
const requestOptions = {
method: "GET",
headers: myHeaders,
redirect: "follow"
};
fetch("https://api.vanta.com/v1/controls?pageSize=10&pageCursor=<string>&frameworkMatchesAny=soc2", requestOptions)
.then((response) => response.text())
.then((result) => console.log(result))
.catch((error) => console.error(error));
const axios = require('axios');
let config = {
method: 'get',
maxBodyLength: Infinity,
url: 'https://api.vanta.com/v1/controls?pageSize=10&pageCursor=<string>&frameworkMatchesAny=soc2',
headers: {
'Accept': 'application/json',
'Authorization': 'Bearer vat_YOUR_TOKEN'
}
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
import requests
url = "https://api.vanta.com/v1/controls?pageSize=10&pageCursor=<string>&frameworkMatchesAny=soc2"
payload = {}
headers = {
'Accept': 'application/json',
'Authorization': 'Bearer vat_YOUR_TOKEN'
}
response = requests.request("GET", url, headers=headers, data=payload)
print(response.text)
List Controls - Example Response
{
"results": {
"pageInfo": {
"endCursor": "c3lzdGVtLW11bHRpcGxlLWF2YWlsYWJpbGl0eS16b25lcw==",
"hasNextPage": true,
"hasPreviousPage": false,
"startCursor": "ZGF0YWJhc2UtcmVwbGljYXRpb24tdXRpbGl6ZWQ="
},
"data": [
{
"id": "database-replication-utilized",
"externalId": "BCD-4",
"name": "Database replication utilized",
"description": "The company's databases are replicated to a secondary data center in real-time. Alerts are configured to notify administrators if replication fails.",
"source": "Vanta",
"domains": [
"BUSINESS_CONTINUITY_&_DISASTER_RECOVERY"
],
"owner": {
"id": "5fc82421a228f6b6f713547d",
"emailAddress": "admin@noprobllama.app",
"displayName": "Admin Admin"
}
},
{
"id": "system-multiple-availability-zones",
"externalId": "BCD-6",
"name": "Production multi-availability zones established",
"description": "The company has a multi-location strategy for production environments employed to permit the resumption of operations at other company data centers in the event of loss of a facility.",
"source": "Vanta",
"domains": [
"BUSINESS_CONTINUITY_&_DISASTER_RECOVERY"
],
"owner": {
"id": "5fc82421a228f6b6f713547d",
"emailAddress": "admin@noprobllama.app",
"displayName": "Admin Admin"
}
}
]
}
}
We'll be using the Control's id
not the externalId
in the next step.
id
not the externalId
in the next step.List Controls - Response Schema
Below is a bullet list explaining each property in the List Controls response body:
results
: Contains the main result data.
results
: Contains the main result data.data
: An array of control objects.
data
: An array of control objects.- Control Object:
-
id
: The unique identifier for the control. -
externalId
: The external identifier for the control. -
name
: The name of the control. -
description
: A brief description of the control. -
source
: The source of the control. -
domains
: An array of domains associated with the control. -
owner
: Information about the owner of the control.
-
Set Control Owner Endpoint - Assign an owner to a control
In this request, we'll be sending a request body containing the UserId of the Vanta User we'd like to make an owner of a Control. We must specify which Control we're targeting in the id
Path variable.
Endpoint:
/controls/:id/set-owner
Path Variables:
- id (
<string>
- Required): The unique identifier for the control. This parameter is necessary to specify which control the request is targeting.
Request Body:
{
"userId": "<string>"
}
Obtain this Id from the People Page in Vanta or via the List People endpoint.
Set Control Owner - Code Example
curl --location 'https://api.vanta.com/v1/controls/database-replication-utilized/set-owner' \
--header 'Content-Type: application/json' \
--header 'Accept: application/json' \
--header 'Authorization: Bearer vat_YOUR_TOKEN' \
--data '{
"userId": "611fd13785dbc71bb89fd401"
}'
const myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
myHeaders.append("Accept", "application/json");
myHeaders.append("Authorization", "Bearer vat_YOUR_TOKEN");
const raw = JSON.stringify({
"userId": "611fd13785dbc71bb89fd401"
});
const requestOptions = {
method: "POST",
headers: myHeaders,
body: raw,
redirect: "follow"
};
fetch("https://api.vanta.com/v1/controls/database-replication-utilized/set-owner", requestOptions)
.then((response) => response.text())
.then((result) => console.log(result))
.catch((error) => console.error(error));
const axios = require('axios');
let data = JSON.stringify({
"userId": "611fd13785dbc71bb89fd401"
});
let config = {
method: 'post',
maxBodyLength: Infinity,
url: 'https://api.vanta.com/v1/controls/database-replication-utilized/set-owner',
headers: {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Bearer vat_YOUR_TOKEN'
},
data : data
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
import requests
import json
url = "https://api.vanta.com/v1/controls/database-replication-utilized/set-owner"
payload = json.dumps({
"userId": "611fd13785dbc71bb89fd401"
})
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Bearer vat_YOUR_TOKEN'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
Set Control Owner - Example Response
{
"id": "database-replication-utilized",
"externalId": "BCD-4",
"name": "Database replication utilized",
"description": "The company's databases are replicated to a secondary data center in real-time. Alerts are configured to notify administrators if replication fails.",
"source": "Vanta",
"domains": [
"BUSINESS_CONTINUITY_&_DISASTER_RECOVERY"
],
"owner": {
"id": "611fd13785dbc71bb89fd401",
"emailAddress": "alejandro@alejandro.com",
"displayName": "Alejandro Ocampo"
}
}
Set Control Owner - Response Schema
Below is a bullet list explaining each property in the Set Control Owner response body:
-
id
: The unique identifier for the control. -
externalId
: The external identifier for the control. -
name
: The name of the control. -
description
: A brief description of the control. -
source
: The source of the control. -
domains
: An array of domains associated with the control. -
owner
: Information about the owner of the control.
We can confirm the Control ownership change when viewing the Control in Vanta:
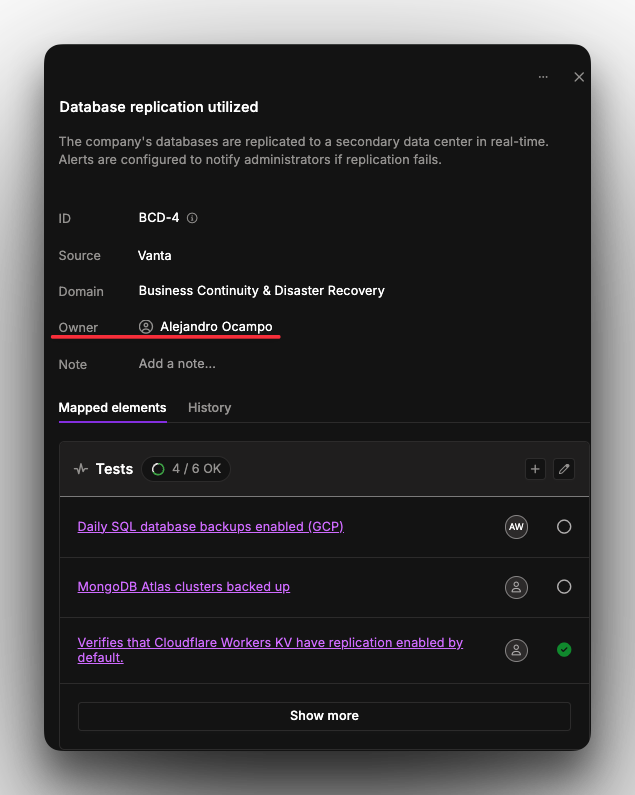
Updated 12 days ago