Auditor application setup
Prerequisites
Only follow this guide if you are a Vanta audit partner interested in using the Vanta API to assist in conducting audits.
You will already need to be registered as an existing Vanta Audit Partner and have an auditor login to successfully complete the rest of this guide.
Navigate to app.vanta.com and login using your auditor email to continue.
Application Creation
- After logging in, you should see the "auditor API" page on the left hand navigation pane. If you do not have this option, please reach out to your Vanta representative to get this resolved.
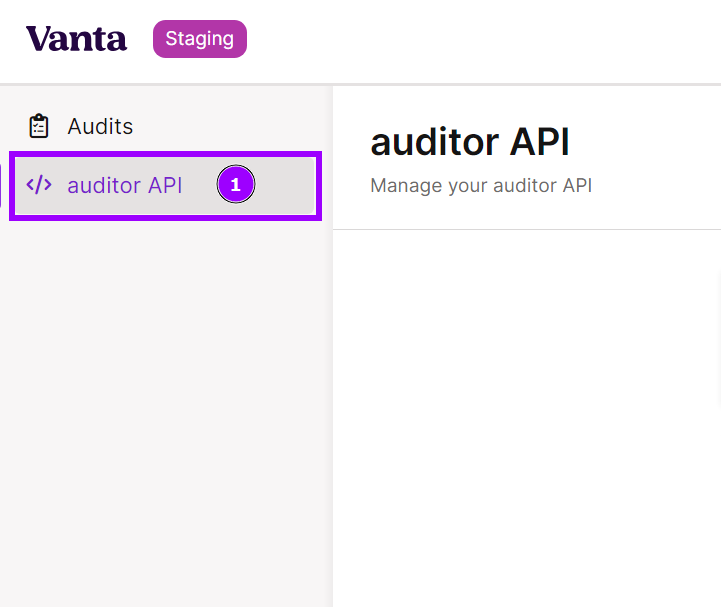
- Create a new application using the "Create" button in the top right.
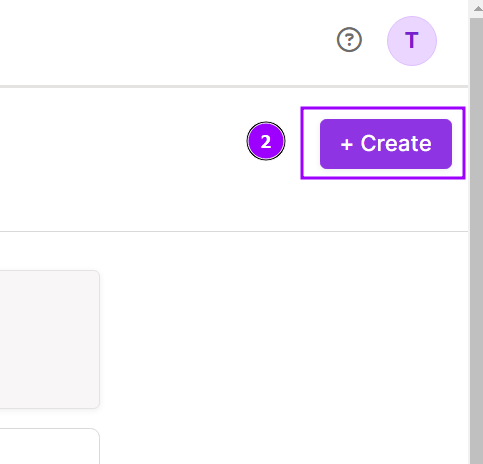
- Give your new application a name and a description and click create.
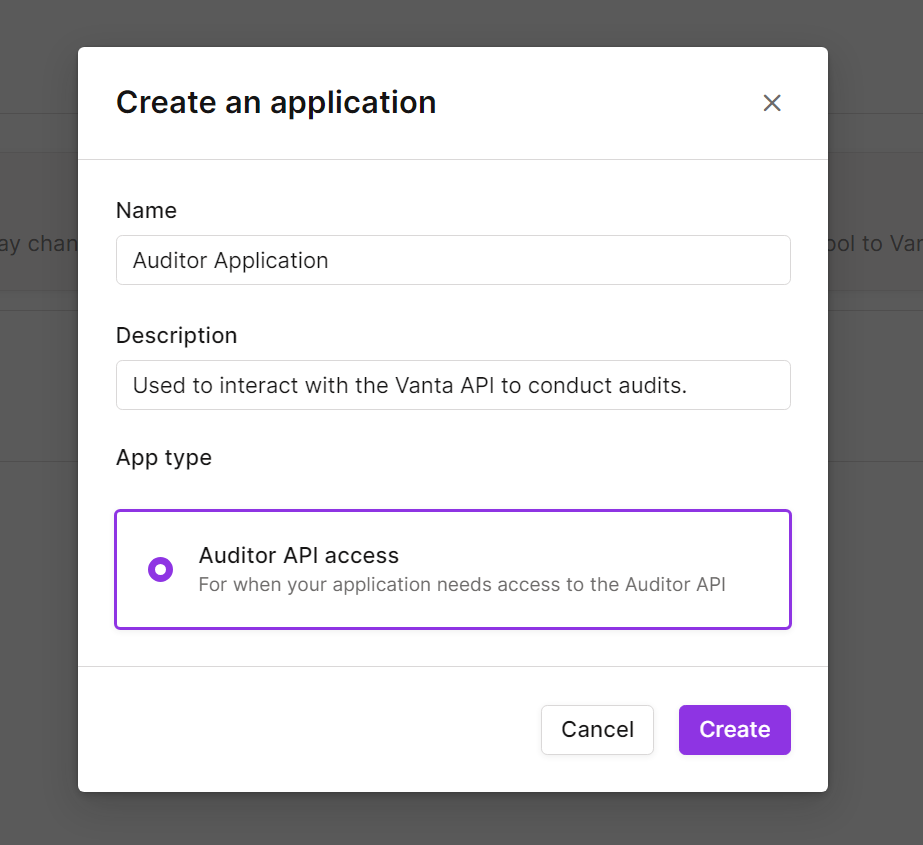
- To successfully authenticate against the Vanta API, you will need a client id and a client secret. Vanta will provide your new application a client id by default, but you will need to click "Generate client secret" to generate the secret value. If your secret ever gets compromised, you can always re-generate a new value here.
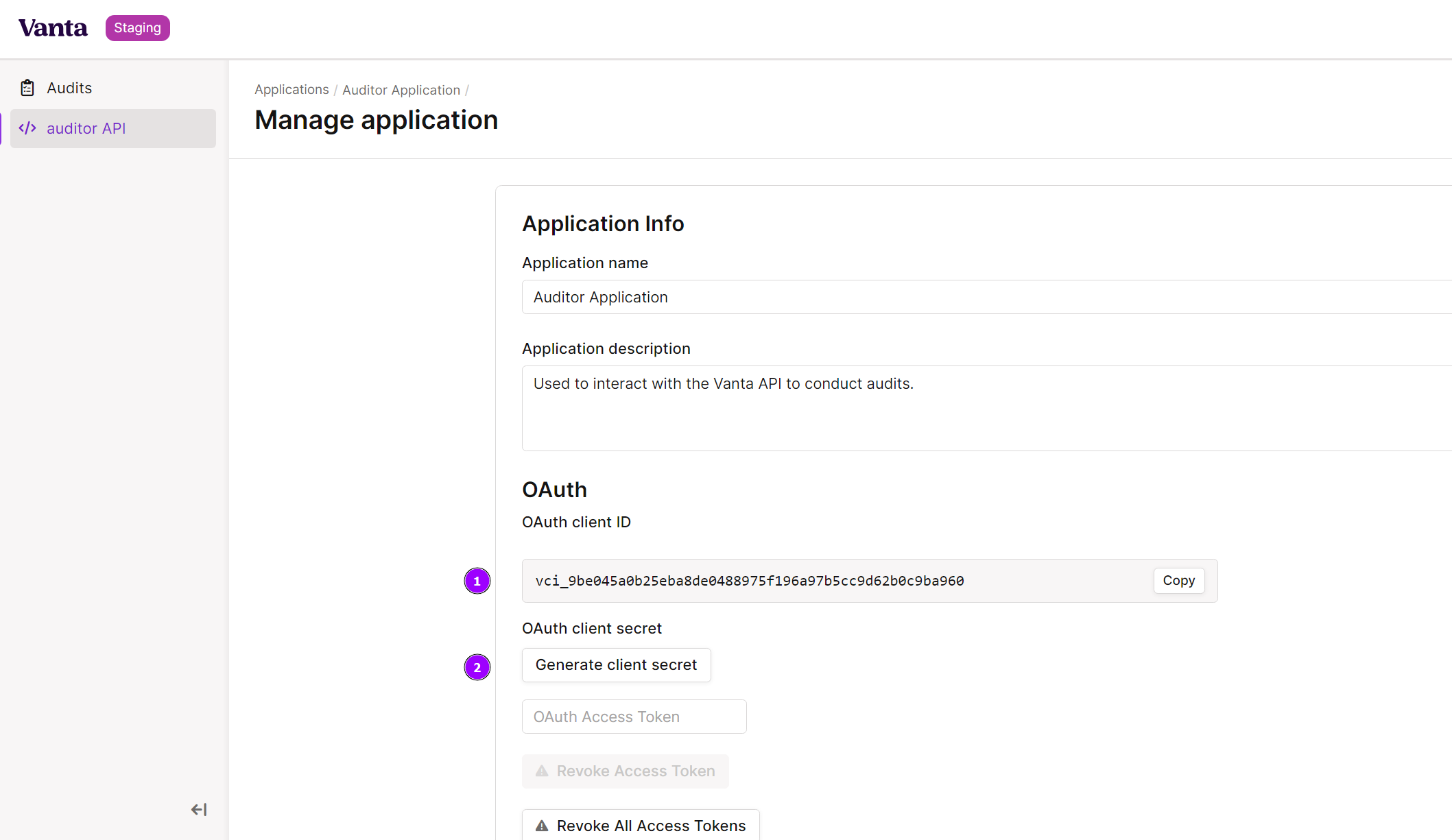
Authentication and Token Retrieval
Now that we have our credentials prepared, the next step is to retrieve our API token with the proper permission scopes. For more information on the available permission scopes, please reference this guide.
- POST to the endpoint https://api.vanta.com/oauth/token
- Header: Content-Type | application/json
- Body:
{ "client_id": "your_client_id", "client_secret": "your_client_secret", "scope": "auditor-api.audit:read auditor-api.audit:write auditor-api.auditor:read auditor-api.auditor:write", "grant_type": "client_credentials" }
Here is an example cURL (replace the client id and secret with the values you generated from previous steps):
curl --location 'https://api.vanta.com/oauth/token' \
--header 'Content-Type: application/json' \
--data '{
"client_id": "your_client_id",
"client_secret": "your_client_secret",
"scope": "auditor-api.audit:read auditor-api.audit:write auditor-api.auditor:read auditor-api.auditor:write",
"grant_type": "client_credentials"
}'
const myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
const raw = JSON.stringify({
"client_id": "vci_TOKEN",
"client_secret": "vcs_f64356_TOKEN",
"scope": "vanta-api.all:read vanta-api.all:write",
"grant_type": "client_credentials"
});
const requestOptions = {
method: "POST",
headers: myHeaders,
body: raw,
redirect: "follow"
};
fetch("https://api.vanta.com/oauth/token", requestOptions)
.then((response) => response.text())
.then((result) => console.log(result))
.catch((error) => console.error(error));
const axios = require('axios');
let data = JSON.stringify({
"client_id": "vci_TOKEN",
"client_secret": "vcs_f64356_TOKEN",
"scope": "vanta-api.all:read vanta-api.all:write",
"grant_type": "client_credentials"
});
let config = {
method: 'post',
maxBodyLength: Infinity,
url: 'https://api.vanta.com/oauth/token',
headers: {
'Content-Type': 'application/json'
},
data : data
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
import requests
import json
url = "https://api.vanta.com/oauth/token"
payload = json.dumps({
"client_id": "vci_TOKEN",
"client_secret": "vcs_f64356_TOKEN",
"scope": "vanta-api.all:read vanta-api.all:write",
"grant_type": "client_credentials"
})
headers = {
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
The response should look like this (where your token is stored in the access_token
property):
{
"access_token": "vat_your_token",
"expires_in": 3599,
"token_type": "Bearer"
}
Note: Your access_token
is only valid for 1 hour but can be refreshed on any cadence you prefer!
Example API Request
You can now use your new API token to authenticate against the other auditor endpoints! It's important to note that you will need an existing active audit to have data return from most of the endpoints. Here is an example cURL to pull a list of existing audits (input your token from the previous call in the authorization header):
curl --location '<https://api.vanta.com/v1/audits?pageSize=10'>
--header 'Accept: application/json'
--header 'Authorization: Bearer your_token_here'
Updated about 2 months ago