Query test results and filter for failing resources
Query test results and filter for failing resources with the Vanta API
Query filtered tests and failing entities
This guide will walkthrough how to query test details and failing test entities using the Vanta API.
In order to query test results and subsequent failing entities, we'll first need to obtain the test's ID. We can get this TestId by querying a list of tests and filtering by parameters like status and category. The other option is to obtain the testId from viewing the test in Vanta. Each test's ID will be located in the URL while viewing a test:
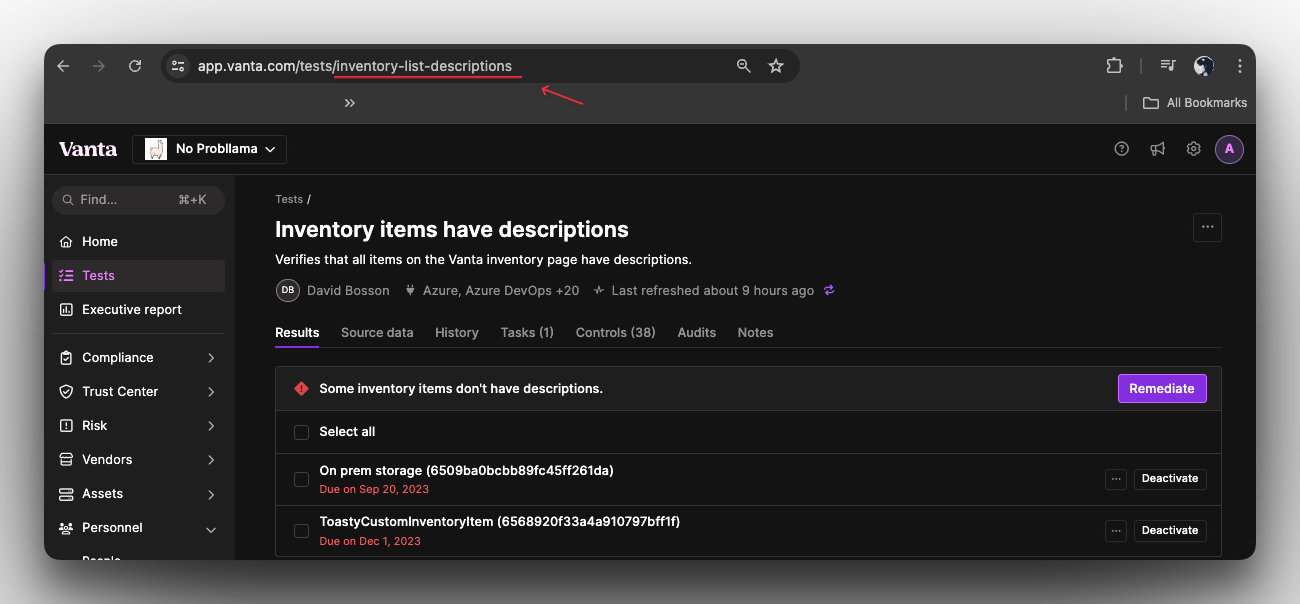
Steps:
- GET a list of tests, filtered by status and category.
- GET a list of test entities by TestId, and filter by entity status.
List Tests Endpoint - Filter by status and category
The List Tests endpoint allows us to query a list of our tests, and filter by several helpful query parameters. We'll use this endpoint to find a test we want to query failing entities on by filtering by test status and category.
Endpoint:
/tests
Query Parameters:
- pageSize (
<number>
): An integer value that specifies the number of resources to return per page. This parameter controls the size of each page in paginated responses. - pageCursor (
<string>
): A string value used to navigate through pages of results. This parameter holds the cursor for the current page, allowing the API to fetch the next set of results. - statusFilter (
ENUM<string>
): Filter tests by test status. Possible values:OK
(Test passed),DEACTIVATED
(Test is deactivated),NEEDS_ATTENTION
(Test failed),IN_PROGRESS
(Test is in progress),INVALID
(Test is invalid),NOT_APPLICABLE
(Test is not applicable). - frameworkFilter (
<string>
): Filter tests by framework. This parameter is used to filter the tests based on a specific framework. - integrationFilter (
<string>
): Filter tests by integration. This parameter is used to filter the tests based on a specific integration. - controlFilter (
<string>
): Filter tests by control id. This parameter is used to filter the tests based on a specific control id. - ownerFilter (
<string>
): Filter tests by owner id. This parameter is used to filter the tests based on a specific owner id. - categoryFilter (
ENUM<string>
): Filter tests by category. Possible values:['ACCOUNTS_ACCESS', 'ACCOUNT_SECURITY', 'ACCOUNT_SETUP', 'COMPUTERS', 'CUSTOM', 'DATA_STORAGE', 'EMPLOYEES', 'INFRASTRUCTURE', 'IT', 'LOGGING', 'MONITORING_ALERTS', 'PEOPLE', 'POLICIES', 'RISK_ANALYSIS', 'SOFTWARE_DEVELOPMENT', 'VENDORS', 'VULNERABILITY_MANAGEMENT']
. - isInRollout (
<boolean>
): Filter tests by rollout status. A test in rollout is an upcoming test that does not have its history tracked yet.
In this request, we'll use the following parameters:
?pageSize=10
&statusFilter=NEEDS_ATTENTION
&categoryFilter=INFRASTRUCTURE
List Tests - Code Example
curl --location 'https://api.vanta.com/v1/tests?pageSize=10&pageCursor=%3Cstring%3E&statusFilter=NEEDS_ATTENTION&categoryFilter=INFRASTRUCTURE' \
--header 'Accept: application/json' \
--header 'Authorization: Bearer vat_YOUR_TOKEN'
const myHeaders = new Headers();
myHeaders.append("Accept", "application/json");
myHeaders.append("Authorization", "Bearer vat_YOUR_TOKEN");
const requestOptions = {
method: "GET",
headers: myHeaders,
redirect: "follow"
};
fetch("https://api.vanta.com/v1/tests?pageSize=10&pageCursor=<string>&statusFilter=NEEDS_ATTENTION&categoryFilter=INFRASTRUCTURE", requestOptions)
.then((response) => response.text())
.then((result) => console.log(result))
.catch((error) => console.error(error));
const axios = require('axios');
let config = {
method: 'get',
maxBodyLength: Infinity,
url: 'https://api.vanta.com/v1/tests?pageSize=10&pageCursor=<string>&statusFilter=NEEDS_ATTENTION&categoryFilter=INFRASTRUCTURE',
headers: {
'Accept': 'application/json',
'Authorization': 'Bearer vat_YOUR_TOKEN'
}
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
import requests
url = "https://api.vanta.com/v1/tests?pageSize=10&pageCursor=<string>&statusFilter=NEEDS_ATTENTION&categoryFilter=INFRASTRUCTURE"
payload = {}
headers = {
'Accept': 'application/json',
'Authorization': 'Bearer vat_YOUR_TOKEN'
}
response = requests.request("GET", url, headers=headers, data=payload)
print(response.text)
List Tests - Example Response
{
"results": {
"pageInfo": {
"endCursor": "aXAtYWNjZXNzLXJ1bGVzLWNsb3VkZmxhcmU=",
"hasNextPage": false,
"hasPreviousPage": false,
"startCursor": "aW52ZW50b3J5LWxpc3QtZGVzY3JpcHRpb25z"
},
"data": [
{
"id": "inventory-list-descriptions",
"name": "Inventory items have descriptions",
"lastTestRunDate": "2024-07-26T13:58:44.518Z",
"latestFlipDate": "2023-07-31T00:17:38.481Z",
"description": "Verifies that all items on the Vanta inventory page have descriptions.\n",
"failureDescription": "Some inventory items don't have descriptions.",
"remediationDescription": "1. Go to the [Inventory list](/inventory).\n2. Ensure that each item in the list has a description.\n\nRecommended: For your cloud resources, we offer a bulk tag option to help manage these resources. Click [here](/inventory?bulk-tags=open) for further instructions on how to use tags to populate inventory metadata.\n\n#### Remediation for Terraform\nAdd the \"VantaDescription\" tag to your resources and set the value to a description of the resource. This information will reflect on the [inventory page](https://app.vanta.com/inventory).\n\n```hcl\nresource \"aws_s3_bucket\" \"data\" {\n bucket = \"data-bucket\"\n\n tags = {\n VantaDescription = \"This bucket stores access logs\"\n }\n}\n```\n\n*Note that the tag key may be different from \"VantaDescription\" if you are utilizing [custom tags](https://app.vanta.com/inventory?bulk-tags=open#compute).",
"version": {
"major": 5,
"minor": 1,
"_id": "66a311700b9be0c02e6206c6"
},
"category": "Infrastructure",
"integrations": [
"aws",
"azure",
"azuredevops",
"gcp",
"addigy",
"bitbucket",
"cloudflare",
"datto",
"digitalocean",
"digitaloceanspaces",
"elastic",
"github",
"gitlab",
"heroku",
"jamf",
"jumpcloud",
"kandji",
"manage_engine",
"microsoft_endpoint_manager",
"mongoatlas",
"netlify",
"ninjaone",
"rippling_mdm",
"simplemdm",
"snowflake",
"supabase",
"vercel",
"workspace_one"
],
"status": "NEEDS_ATTENTION",
"deactivatedStatusInfo": {
"isDeactivated": false,
"deactivatedReason": null,
"lastUpdatedDate": null
},
"remediationStatusInfo": {
"status": "OVERDUE",
"soonestRemediateByDate": "2023-09-20T15:11:59.765Z",
"itemCount": 2
},
"owner": {
"id": "62fb92241328a26f5d3fa536",
"emailAddress": "[email protected]",
"displayName": "David Bosson"
}
},
{
"id": "inventory-list-owners",
"name": "Inventory items have active owners",
"lastTestRunDate": "2024-07-26T23:20:42.307Z",
"latestFlipDate": "2023-07-31T00:03:33.198Z",
"description": "Verifies that all items on the Vanta inventory page have been assigned owners, and that these owners are still active employees.\n",
"failureDescription": "Some inventory items don't have owners, or their owners are not active employees.",
"remediationDescription": "### For Cloud Resources\n\nGo to the [inventory list](/inventory) and ensure that each item in the list has an owner.\n\nRecommended: We offer a bulk tag option to help manage these resources. Click [here](/inventory?bulk-tags=open) for further instructions on how to use tags to assign inventory owners.\n\n### For computers managed by the Vanta Agent\n\nEnsure that each device has an active owner. You should change device owners from the inventory page.\n\n- Click [here](/inventory?owner=NO_OWNER_ID#employee-computers-agent) to check for computers managed by the Vanta Agent without an owner.\n- Devices assigned to former employees need to be reassigned. Click [here](/inventory?owner=FORMER_EMPLOYEE_OWNERS_ID#employee-computers-agent) to check for computers managed by the Vanta Agent assigned to former employees.\n\n### For computers managed by an integrated MDM\n\nEnsure that each device has an active owner. You should change the assigned owner in the MDM platform.\n\n- Click [here](/inventory?owner=NO_OWNER_ID#employee-computers-mdm) to check for computers managed by an MDM (other than the Vanta Agent) without an owner.\n- Devices assigned to former employees need to be reassigned. Click [here](/inventory?owner=FORMER_EMPLOYEE_OWNERS_ID#employee-computers-mdm) to check for computers managed by an MDM (other than the Vanta Agent) assigned to former employees.\n\nIf the test is still failing after following the steps above, please refer to our [help article for this test here](https://help.vanta.com/hc/en-us/articles/15657151148436-Resolve-Inventory-items-have-owners-Test).\n\n#### Remediation for Terraform\nAdd the \"VantaOwner\" tag to your resources and set the value to an email address for a currently active Vanta user. This information will reflect on the [inventory page](https://app.vanta.com/inventory).\n\n```hcl\nresource \"aws_s3_bucket\" \"data\" {\n bucket = \"data-bucket\"\n\n tags = {\n VantaOwner = \"[email protected]\"\n }\n}\n```\n\n*Note that the tag key may be different from \"VantaOwner\" if you are utilizing [custom tags](https://app.vanta.com/inventory?bulk-tags=open#compute).",
"version": {
"major": 5,
"minor": 0,
"_id": "66a311710b9be0c02e620804"
},
"category": "Infrastructure",
"integrations": [
"aws",
"azure",
"azuredevops",
"gcp",
"addigy",
"bitbucket",
"cloudflare",
"datto",
"digitalocean",
"digitaloceanspaces",
"elastic",
"github",
"gitlab",
"heroku",
"jamf",
"jumpcloud",
"kandji",
"manage_engine",
"microsoft_endpoint_manager",
"mongoatlas",
"netlify",
"ninjaone",
"rippling_mdm",
"simplemdm",
"snowflake",
"supabase",
"vercel",
"workspace_one"
],
"status": "NEEDS_ATTENTION",
"deactivatedStatusInfo": {
"isDeactivated": false,
"deactivatedReason": null,
"lastUpdatedDate": null
},
"remediationStatusInfo": {
"status": "OVERDUE",
"soonestRemediateByDate": "2023-09-20T15:12:00.092Z",
"itemCount": 8
},
"owner": {
"id": "64992d9c01bf8bd6638dfe40",
"emailAddress": "[email protected]",
"displayName": "Alex González"
}
}
]
}
}
List Tests - Response Schema
Below is a bullet list explaining each property in the List Tests response body:
results
: Contains the main result data.
results
: Contains the main result data.data
: An array of test objects.
data
: An array of test objects.- Test Object:
-
id
: A unique identifier for the test. -
name
: The name of the test. -
lastTestRunDate
: The date and time when the test was last run. -
latestFlipDate
: The date and time when the test status last changed. -
description
: A brief description of the test. -
failureDescription
: A description of the test failure. -
remediationDescription
: Instructions on how to remediate the test failure. -
version
: Version information of the test. -
category
: The category of the test. -
integrations
: An array of integration identifiers associated with the test. -
status
: The current status of the test (e.g., "NEEDS_ATTENTION"). -
deactivatedStatusInfo
: Information about the deactivated status of the test. -
remediationStatusInfo
: Information about the remediation status of the test. -
owner
: Information about the owner of the test.
-
Get Test Entities Endpoint - Filter for failing Entities
With the Vanta Test's ID, we can query a tests entities. Filtering with the entityStatus parameter will return a list of test entities of that status on the test.
Endpoint:
/tests/:testId/entities
Query Parameters:
-
entityStatus (
ENUM<string>
): The status of the entities to return. Defaults toFAILING
.
Possible values:FAILING
,DEACTIVATED
. -
pageSize (
10
): An integer value that specifies the number of resources to return per page. This parameter controls the size of each page in paginated responses. -
pageCursor (
<string>
): A string value used to navigate through pages of results. This parameter holds the cursor for the current page, allowing the API to fetch the next set of results.
Path Variables:
- testId (
<string>
- Required): The unique identifier for the test. This variable is necessary to specify which test the request is targeting.
Get Test Entities - Code Example
curl --location 'https://api.vanta.com/v1/tests/inventory-list-descriptions/entities?entityStatus=FAILING&pageSize=10&pageCursor=%3Cstring%3E' \
--header 'Accept: application/json' \
--header 'Authorization: Bearer vat_YOUR_TOKEN'
const myHeaders = new Headers();
myHeaders.append("Accept", "application/json");
myHeaders.append("Authorization", "Bearer vat_YOUR_TOKEN");
const requestOptions = {
method: "GET",
headers: myHeaders,
redirect: "follow"
};
fetch("https://api.vanta.com/v1/tests/inventory-list-descriptions/entities?entityStatus=FAILING&pageSize=10&pageCursor=<string>", requestOptions)
.then((response) => response.text())
.then((result) => console.log(result))
.catch((error) => console.error(error));
const axios = require('axios');
let config = {
method: 'get',
maxBodyLength: Infinity,
url: 'https://api.vanta.com/v1/tests/inventory-list-descriptions/entities?entityStatus=FAILING&pageSize=10&pageCursor=<string>',
headers: {
'Accept': 'application/json',
'Authorization': 'Bearer vat_YOUR_TOKEN'
}
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
import requests
url = "https://api.vanta.com/v1/tests/inventory-list-descriptions/entities?entityStatus=FAILING&pageSize=10&pageCursor=<string>"
payload = {}
headers = {
'Accept': 'application/json',
'Authorization': 'Bearer vat_YOUR_TOKEN'
}
response = requests.request("GET", url, headers=headers, data=payload)
print(response.text)
Get Test Entities - Example Response
{
"results": {
"pageInfo": {
"endCursor": "NjQwNWY5ZDc3MjRjZDJiMGYxMzg5MGY0",
"hasNextPage": false,
"hasPreviousPage": false,
"startCursor": "NjMyYjkyZjY5NDM0ODE0YzE1NDllNjY4"
},
"data": [
{
"id": "String-632b92f69434814c1549e668",
"entityStatus": "FAILING",
"displayName": "632b92f69434814c1549e668",
"responseType": "Identifier",
"deactivatedReason": null,
"lastUpdatedDate": "2023-06-06T17:18:18.290Z",
"createdDate": "2022-10-24T20:55:24.408Z"
},
{
"id": "String-63c58dc8d936e30c598b374e",
"entityStatus": "FAILING",
"displayName": "63c58dc8d936e30c598b374e",
"responseType": "Identifier",
"deactivatedReason": null,
"lastUpdatedDate": "2023-06-06T17:18:18.290Z",
"createdDate": "2023-01-16T17:47:58.948Z"
},
{
"id": "String-63e68cdf5a6cd6d4e2797224",
"entityStatus": "FAILING",
"displayName": "63e68cdf5a6cd6d4e2797224",
"responseType": "Identifier",
"deactivatedReason": null,
"lastUpdatedDate": "2023-06-06T17:18:18.290Z",
"createdDate": "2023-02-10T18:28:52.985Z"
},
{
"id": "String-6405f9d5b821bf4249c01efc",
"entityStatus": "FAILING",
"displayName": "6405f9d5b821bf4249c01efc",
"responseType": "Identifier",
"deactivatedReason": null,
"lastUpdatedDate": "2023-06-06T17:18:18.290Z",
"createdDate": "2023-03-06T14:34:04.808Z"
},
{
"id": "String-6405f9d7724cd2b0f13890f4",
"entityStatus": "FAILING",
"displayName": "6405f9d7724cd2b0f13890f4",
"responseType": "Identifier",
"deactivatedReason": null,
"lastUpdatedDate": "2023-06-06T17:18:18.290Z",
"createdDate": "2023-03-06T14:34:04.808Z"
}
]
}
}
Get Test Entities - Response Schema
Below is a bullet list explaining each property in the List Entities response body:
results
: Contains the main result data.
results
: Contains the main result data.data
: An array of entity objects.
data
: An array of entity objects.- Entity Object:
-
id
: A unique identifier for the entity. -
entityStatus
: The status of the entity, e.g., "FAILING". -
displayName
: The display name of the entity. -
responseType
: The type of the entity, e.g., "Identifier". -
deactivatedReason
: The reason for deactivation, if applicable (null if not deactivated). -
lastUpdatedDate
: The date and time when the entity was last updated. -
createdDate
: The date and time when the entity was created.
-
Updated 5 months ago